📌 Debounce & Throttle
: 자주 사용되는 이벤트나 함수의 실행되는 빈도를 줄여서, 성능의 유리함을 가져오기 위한 개념
- 사용자의 의도와 무관한 요청을 줄이기 위한 기능 (input 입력 이벤트, API로 데이터를 가져오는 이벤트 등)
- 스크롤, 윈도우 리사이즈, 마우스 이동 등의 빈번한 이벤트에서 성능을 최적화하는 데 유용
- 이벤트가 과도하게 발생하는 것을 제한
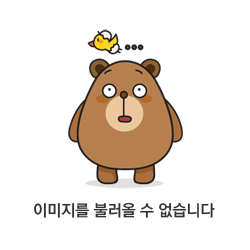
✅ Debounce
: 입력 주기가 끝남과 동시에 이벤트를 호출한다.
- 같은 이벤트가 반복되게 발생하는 경우, 반복적으로 발생하던 이벤트가 일정 시간동안 다시 발생하지 않으면 콜백 함수를 실행
- 아무리 많은 이벤트가 발생해도 모두 무시하고 특정 시간 사이에 어떤 이벤트도 발생하지 않았을 때 딱 한번만 마지막 이벤트를 발생시킨다.
- 키워드 검색, 자동완성 기능, 사용자 창 크기 조정(윈도우 리사이즈)
function debounce(callback, limit = 100) {
let timeout
return function(...args) {
clearTimeout(timeout)
timeout = setTimeout(() => {
callback.apply(this, args)
}, limit)
}
}
설치
npm install debounce
사용
// debounce 불러오기
import debounce from 'debounce';
function resize() {
console.log('height', window.innerHeight);
console.log('width', window.innerWidth);
}
// debounce 사용하기
window.onresize = debounce(resize, 200);
예제
- 입력 이벤트 처리
// debounce 모듈 가져오기
const debounce = require('debounce');
// 입력 값이 변경될 때 호출되는 함수
function handleInputChange(event) {
console.log('Input value:', event.target.value);
}
// 300ms 지연된 debounce 함수
const debouncedHandleInputChange = debounce(handleInputChange, 300);
// HTML 문서에서 input 요소 찾기
const inputElement = document.getElementById('myInput');
// 'input' 이벤트가 발생할 때마다 debouncedHandleInputChange 함수를 호출
inputElement.addEventListener('input', debouncedHandleInputChange);
✅ Throttle
: 입력 주기를 방해하지 않고, 일정한 시간 간격으로 이벤트를 호출한다.
- 같은 이벤트가 반복되게 발생하는 경우, 일정 시간 간격으로 콜백 함수를 실행
- 스크롤 이벤트
- 스크롤 이벤트를 발생시키면, throttle을 사용한 함수는 매번 스크롤 이벤트가 발생할 때마다 실행되지 않고 정해진 간격으로만 실행된다.
function throttle(callback, limit = 100) {
let waiting = false
return function() {
if(!waiting) {
callback.apply(this, arguments)
waiting = true
setTimeout(() => {
waiting = false
}, limit)
}
}
}
설치
npm i lodash.throttle
사용
// lodash의 메서드 가져오기
const _ = require('lodash');
function handleScroll() {
console.log('스크롤 이벤트 발생!');
}
// throttle 사용
const throttledScroll = _.throttle(handleScroll, 1000);
window.addEventListener('scroll', throttledScroll);
예제
- 윈도우 리사이즈 이벤트 & 마우스 이동 이벤트 처리
// lodash의 throttle 함수 가져오기
const _ = require('lodash');
// 윈도우 리사이즈 이벤트 제어 함수
const throttledResize = _.throttle(function() {
console.log('윈도우 크기 변경 이벤트 발생!');
}, 500);
// 윈도우 리사이즈 이벤트에 throttledResize 함수를 추가
window.addEventListener('resize', throttledResize);
// 마우스 이동 이벤트 제어 함수
const throttledMouseMove = _.throttle(function(event) {
console.log(`마우스 위치: ${event.clientX}, ${event.clientY}`);
}, 200);
// 마우스 이동 이벤트에 throttledMouseMove 함수를 추가
window.addEventListener('mousemove', throttledMouseMove);
debounce
Delay function calls until a set time elapses after the last invocation. Latest version: 2.1.0, last published: 2 months ago. Start using debounce in your project by running `npm i debounce`. There are 1847 other projects in the npm registry using debounce
www.npmjs.com
lodash.throttle
The lodash method `_.throttle` exported as a module.. Latest version: 4.1.1, last published: 8 years ago. Start using lodash.throttle in your project by running `npm i lodash.throttle`. There are 3468 other projects in the npm registry using lodash.throttl
www.npmjs.com
Lodash
_.defaults({ 'a': 1 }, { 'a': 3, 'b': 2 });_.partition([1, 2, 3, 4], n => n % 2);DownloadLodash is released under the MIT license & supports modern environments. Review the build differences & pick one that’s right for you.InstallationIn
lodash.com
Debouncing And Throttling Explained Through Examples | CSS-Tricks
The following is a guest post by David Corbacho, a front end engineer in London. We've broached this topic before, but this time, David is going to drive the
css-tricks.com
(JavaScript) 쓰로틀링과 디바운싱
안녕하세요. 이번 시간에는 쓰로틀링(throttling)과 디바운싱(debouncing)에 대해 알아보겠습니다. 원래 예정에 없던 강좌이지만 요청을 받았기 때문에 써봅니다. 프로그래밍 기법 중 하나입니다(아니
www.zerocho.com
Throttle, Debounce 개념 잡기
Rx Java를 사용하다가 접한 개념인데 정리하지 않으면 차이점이 햇갈릴거 같아서 글로 남긴다. 리서치를 해보니 전반적으로 사용되는 기술인듯 하다. (예제는 웹쪽이 압도적으로 많았다.)
medium.com
LinkedIn SleekSky 페이지: 𝗗𝗲𝗯𝗼𝘂𝗻𝗰𝗶𝗻𝗴 𝘃𝘀. 𝗧𝗵𝗿𝗼𝘁𝘁𝗹𝗶𝗻𝗴 in Java
𝗗𝗲𝗯𝗼𝘂𝗻𝗰𝗶𝗻𝗴 𝘃𝘀. 𝗧𝗵𝗿𝗼𝘁𝘁𝗹𝗶𝗻𝗴 in JavaScript - Understanding the Difference, Their Utilities, and When to Use Which? Debouncing and…
www.linkedin.com